agniusm
1 MW
Hi, I have little experience in electronics and even a lot less in programming so this part is the hardest but never the less i would like to try and build myself ebike gauge.
i will be using three sensors, first for voltage, voltage divider, second - current sensor, will be using 100A allegro bidirectional sensor and third, hall effect sensor from hub motor.
i would like to have 4 values to be displayed:
Kilometers left on battery (Which would be calculated on averaging the draw);
Power draw in amps or watts as well as power put in to the battery on regeneration; (Half gimmick half useful for adjusting driving style)
Speed;
Odometer with stored distance in eeprom.
i came up with this layout:
My screen is 128x64 transflective with white backlight:
https://www.youtube.com/watch?v=vBfRDwSbFQg
i chose this LCD for its visibility in direct sunlight:
[youtube]ZLQiJjm7JbI[/youtube]
The driver is ST7565P:
In u8glib using:
greatly appreciated.
So far i have implemented font i would like to use:
After some correction:
and another font just to have few:
Here is speed imitation animation:
[youtube]15tuK5SUqnM[/youtube]
Basically i am after simple, clean, sleek, classy cycle computer with basic functionality implemented, open source for end user. Perhaps i am over my head with this but one must try
I would like some help from community with getting this device done which then be available to buy and modify
I would like it to be milled from solid block aluminum, phone like tinted glass over it, 2 buttons for later implementation.
promising nothing yet but it might end up something.
P.S. Hope this in the right section of the forum.
i will be using three sensors, first for voltage, voltage divider, second - current sensor, will be using 100A allegro bidirectional sensor and third, hall effect sensor from hub motor.
i would like to have 4 values to be displayed:
Kilometers left on battery (Which would be calculated on averaging the draw);
Power draw in amps or watts as well as power put in to the battery on regeneration; (Half gimmick half useful for adjusting driving style)
Speed;
Odometer with stored distance in eeprom.
i came up with this layout:
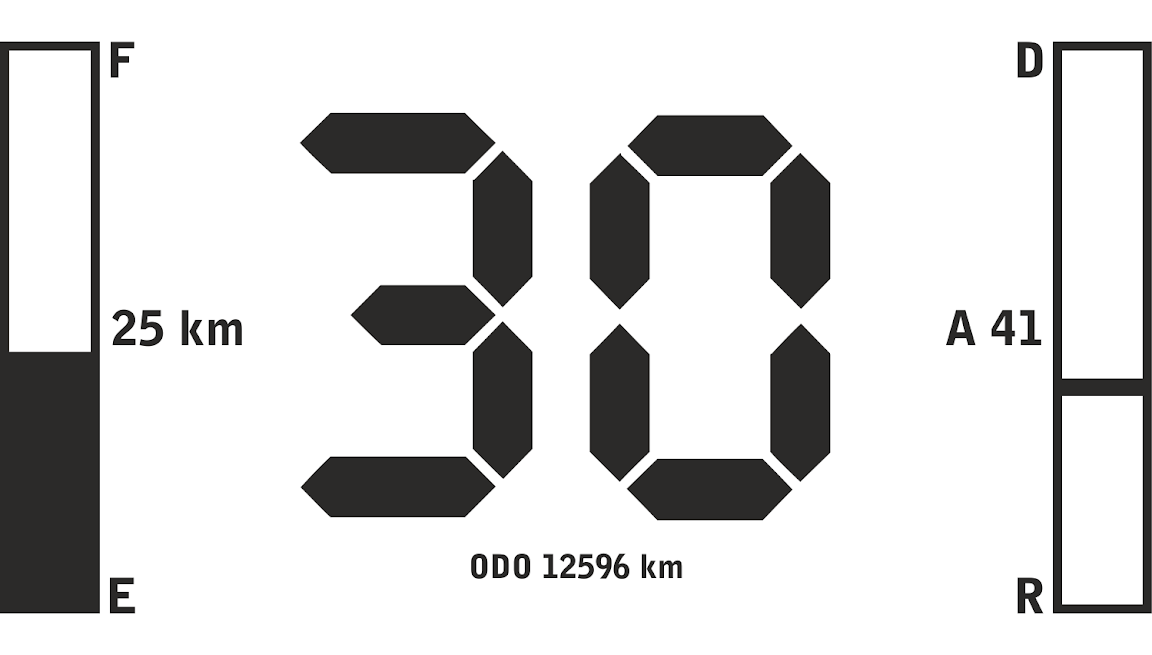
My screen is 128x64 transflective with white backlight:
https://www.youtube.com/watch?v=vBfRDwSbFQg
i chose this LCD for its visibility in direct sunlight:
[youtube]ZLQiJjm7JbI[/youtube]
The driver is ST7565P:
In u8glib using:
Need help with programming the LCD to display correct info and tieing values from input to display output. It could be separate components and i think i could put all together and arrange as i require if there are some comments in the codeU8GLIB_64128N u8g(13, 11, 10, 9, 8);
greatly appreciated.
So far i have implemented font i would like to use:
After some correction:
and another font just to have few:
Here is speed imitation animation:
[youtube]15tuK5SUqnM[/youtube]
Basically i am after simple, clean, sleek, classy cycle computer with basic functionality implemented, open source for end user. Perhaps i am over my head with this but one must try
I would like some help from community with getting this device done which then be available to buy and modify
I would like it to be milled from solid block aluminum, phone like tinted glass over it, 2 buttons for later implementation.
promising nothing yet but it might end up something.
P.S. Hope this in the right section of the forum.